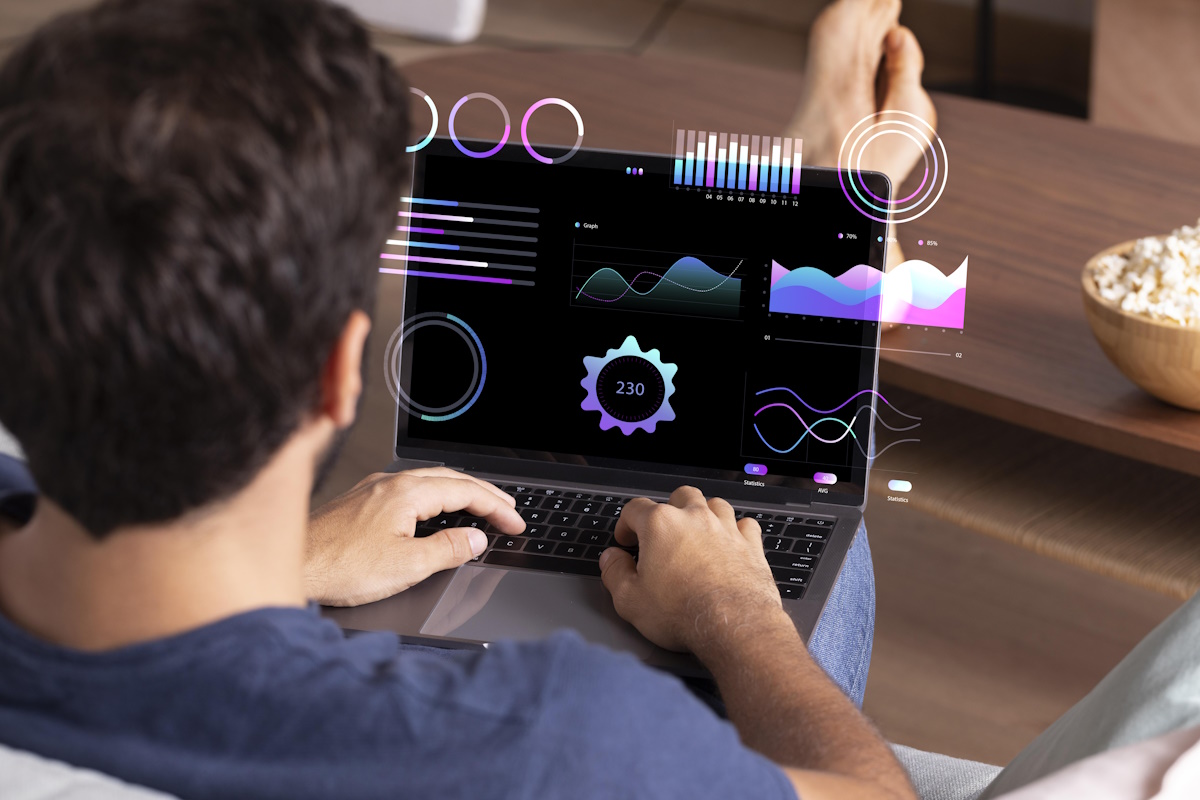
Optimizing Performance in ReactJS Applications
ReactJS is a popular framework known for its efficiency and flexibility in building interactive user interfaces. However, as your application grows in complexity, it’s imperative to ensure it remains performant and responsive. Here are some strategies to optimize performance in ReactJS applications.
Quick Snapshot
Code Optimization Techniques in ReactJS
Code optimization is an effective strategy to improve the performance of a ReactJS application. These techniques not only make the application more efficient but also increase its speed and responsiveness. Below are some of the ways to optimize your ReactJS code:
- Component Optimization: Break your application into smaller, reusable components to make your code cleaner and easier to maintain. Also, avoid unnecessary re-rendering of components using React’s PureComponent or shouldComponentUpdate method.
- Lazy Loading: Consider using React’s lazy function to load components only when they are needed. This can greatly reduce the initial load time of your application.
- Memoization: Use memoization to prevent expensive, repetitive computations. React’s useMemo and useCallback hooks can be useful for this.
- Virtualization: If you are rendering large lists, consider using a technique called virtualization. Libraries like ‘react-window’ provide components that only render items that are currently visible, improving performance.
- Using Production Build: Ensure you are using the production build of React, not the development build, for deploying applications. The production build is optimized for performance and smaller in size.
By employing these techniques, developers can greatly enhance the speed and efficiency of their ReactJS applications.
Virtual DOM
React’s Virtual DOM is a vital feature that enhances application performance. It allows React to keep a lightweight copy of the real DOM in memory, and when the state of an object changes, React first performs an operation on the Virtual DOM. Then, it compares the new Virtual DOM with a pre-update version and finally updates the real DOM, leading to minimal and efficient update operations.
Lazy Loading
Utilizing lazy loading can significantly improve loading time and overall performance. React’s `React.lazy()` function lets you render a dynamic import as a regular component. It automatically loads the bundle containing the component when it’s required.
Memoization
Memoization is a technique used to speed up applications by storing the results of expensive function calls and reusing the results when the same inputs occur again. React provides a built-in hook `React.useMemo()` that will only recompute the memoized value when one of the dependencies has changed, preventing unnecessary renderings.
PureComponent and shouldComponentUpdate
`React.PureComponent` can lead to a performance boost as it prevents unnecessary re-renders. It does a shallow comparison of state and props to decide if the component should update. Similarly, `shouldComponentUpdate` lifecycle method allows control over when re-rendering should occur, further optimizing the performance.
Importance of Performance Optimization
Performance optimization is a crucial aspect of application development that ensures user satisfaction. A well-optimized ReactJS application provides a swift, smooth user experience, leading to higher engagement and retention rates.
- User Experience: Fast and efficient applications provide a better user experience. A slow app can frustrate users, leading them to abandon it, and impacting user retention and engagement.
- SEO Ranking: Google considers page load speed as a key ranking factor. Fast-loading apps are more likely to appear in the top search results, increasing visibility and attracting more users.
- Efficient Resource Use: Optimized applications make better use of system resources. They consume less memory and processing power, which can be crucial for users with low-end devices or slow internet connections.
- Scalability: Performance optimization ensures that your application can handle a large number of users or requests without slowing down, vital for the scalability of the application.
- Reduced Costs: By optimizing performance, we can reduce the need for more server capacity to handle the load, which can significantly decrease operational and hosting costs.
Importance of Measuring Performance
Measuring performance is an essential part of the optimization process in ReacJS web development. Without measurement, it’s impossible to identify bottlenecks and know precisely where to focus optimization efforts.
- Bottleneck Identification: By measuring performance, we can zero in on the areas which slow down our application. These performance bottlenecks, once identified, can be improved or eliminated, leading to a more smoothly operating application.
- Goal Setting & Tracking: Performance metrics allow developers to set tangible, measurable goals for optimization efforts. Once these targets are set, tracking changes in performance becomes possible, providing continuous feedback on the effectiveness of optimization strategies.
- User Experience Evaluation: Measuring performance helps in evaluating the user experience from a technical standpoint. This data can provide insights into how users interact with the app, revealing areas where the user experience can be improved.
- Informed Decision Making: Performance measurements provide valuable data that can inform key decisions. For example, it can guide decisions about when to optimize, what to optimize, and the cost-benefit analysis of different optimization strategies.
- Competitive Advantage: If we have an accurate understanding of our app’s performance, it can offer a competitive advantage. It can help identify opportunities for improvement that competitors may overlook, leading to a better, faster, and more efficient app.
To conclude, optimizing performance in ReactJS applications is crucial to delivering a smooth user experience. By implementing these strategies and keeping an eye on your application’s performance, you can ensure your applications are efficient and user-friendly. Remember to always measure before optimizing, and focus your efforts where they will make the most significant impact.


Average Rating