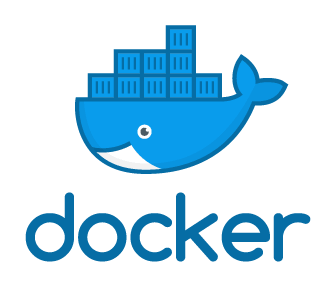
How to Build and run your app with Compose
Docker Compose tool is used to define and start running multi-container Docker applications. Configuration is as easy, there would be a YAML file to configure your application’s services/networks/volumes, etc., Then, with a single command, you can create and start all the services from the compose configuration.
Here are the key steps :
- Define
Dockerfile
for your app’s environment. - Define
docker-compose.yml
for the services that make up your app services. - Run
docker-compose up
and Compose starts and runs your entire app.
This quickstart assumes basic understanding of Docker concepts, please refer earlier posts for understanding on Docker & how to install and containerize applications.In this post,we can look at how to use existing Docker compose file and define services.
Key Features of Docker Compose
- When you define Compose file,you can use project name to isolate environments from each other. This could be useful in cases like creating multiple copies of a single environment or segregate the builds by unique build number.
- Compose caches the configuration used to create a container. When you restart a service that has not changed, Compose re-uses the existing containers.It means that you can make changes to your environment very quickly.
- Compose preserves all volumes used by your services. When
docker-compose up
runs, if it finds any containers from previous runs, it copies the volumes from the old container to the new container. This process ensures that any data you’ve created in volumes isn’t lost. - Compose supports variables in the Compose file. You can use these variables to customize your composition for different environments, or different users.
In the next section,we can look at how define services in compose file for sample application with Angular as front end,Spring Boot as API and Postgres as Database.
Quick Snapshot
#1.Definition of Docker files
We have already defined below docker files in the previous posts here,here & here. Create new project directory and copy all dockerfiles to this folder.
Angular Application Docker file
FROM httpd:2.4 #copy angular dist folder to container COPY dist/ /usr/local/apache2/htdocs/ #copy htaccess and httpd.conf to container COPY .htaccess /usr/local/apache2/htdocs/ COPY httpd.conf /usr/local/apache2/conf/httpd.conf #change permissions RUN chmod -R 755 /usr/local/apache2/htdocs/ #expose port EXPOSE 4200
Spring Boot API Docker file
FROM openjdk:8-jre WORKDIR / #add required jars ADD spring-boot-rest-postgresql-0.0.1-SNAPSHOT.jar spring-boot-rest-postgresql-0.0.1-SNAPSHOT.jar #expose port EXPOSE 8080 #cmd to execute ENTRYPOINT ["java","-Djava.security.egd=file:/dev/./urandom","-jar","/spring-boot-rest-postgresql-0.0.1-SNAPSHOT.jar"]
Database Docker file
FROM postgres:9.5 #init.sql to create database COPY init.sql /docker-entrypoint-initdb.d/ # Adjust PostgreSQL configuration to accept remote connections RUN echo "host all all 0.0.0.0/0 md5" >> /var/lib/postgresql/data/pg_hba.conf RUN echo "listen_addresses='*'" >> /var/lib/postgresql/data/postgresql.conf # expose port EXPOSE 5432
Now that we have dockerfiles ready for all of the application,next step is to define compose file.
#2.Define services in Docker compose file
Create a file called docker-compose.yml
in your project directory and paste the following:
version: '3' services: ui: build: context: . dockerfile: UIDockerfile ports: - '4200:4200' networks: - samplenet links: - 'api:api' api: build: context: . dockerfile: AppDockerfile ports: - '8080:8080' depends_on: - db - rabbitmq networks: - samplenet links: - 'db:db' db: build: context: . dockerfile: DBDockerfile volumes: - 'postgresdb:/var/lib/postgresql/data' environment: POSTGRES_USER: postgres POSTGRES_PASSWORD: postgres POSTGRES_DB: testdb ports: - '5432:5432' healthcheck: test: - CMD-SHELL - 'pg_isready -U postgres' interval: 10s timeout: 5s retries: 5 networks: - samplenet rabbitmq: image: 'rabbitmq:3.5.3-management' container_name: rabbitmq2 ports: - '5672:5672' - '15672:15672' networks: - samplenet networks: samplenet: null volumes: postgresdb: {}
Above compose file defines 4 services as below:
ui
: this is for angular application,it uses an image that’s built from the UIDockerfile in the current directory and forwards the exposed port4200
on the container to port4200
on the host machine.api
: this is for spring boot application,it uses an image that’s built from the AppDockerfile in the current directory and forwards the exposed port8080
on the container to port8080
on the host machine. Note this is dependent ondb
andrabbitmq
services.db
: this is for postgresdb application,it uses an image that’s built from the DBDockerfile in the current directory and forwards the exposed port5432
on the container to port5432
on the host machine.rabbitmq
: Usesrabbitmq:3.5.3-management
public image from Dockerhub registry and uses ports5672,15672
- All the above services uses
samplenet
network and - Persistent volume
postgresdb
definition is fordb
service - Added
healthcheck
section for db service to keep tab on the health of the database depends_on
denotes the service dependencies. When you start the services, compose would start the dependent services as well.
#3.Build and run your app with Compose
In the current project directory, run docker-compose up
to start the application. Compose pulls all the required Docker image, builds an image for your code, and starts the services you defined.


You can stop the application, either by running docker-compose down
from within your project directory or by hitting CTRL+C in the original terminal where you started the app.
To apply patches/updates to any of application container(s) they can be stopped using below command
docker-compose stop <service name>
or if you want to force stop then use below command
docker-compose kill <service name>
To bring up service back online, use below command
docker-compose start <service name>
If you don’t want to compile dependencies, use below command
docker-compose up -d --no-deps <service name>
--no-deps
will not start linked services
-d
Detached mode
If there are existing containers for a service, and the service’s configuration or image was changed after the container’s creation, docker-compose up
command picks up the changes by stopping and recreating the containers.
Congrats! today we have learnt how to build and run your application using Docker compose utility.
Like this post? Don’t forget to share it!
Useful Resources:
- Official documentation as a reference to understand any command.
- Docker build reference
- Docker run reference
- Best Practices article on writing Docker files.
- Test your knowledge on Dockerfile.
- Full list of Compose commands
- Compose configuration file reference
- Monitoring Docker containers using Prometheus + cAdvisor + Grafana
- How to aggregate Docker Container logs and analyse with ELK stack ?
- Implementing secure containers using gVisor+Docker tutorial


Average Rating