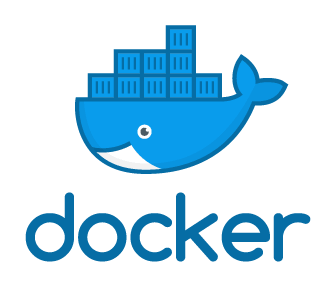
Docker tutorial – Build Docker image for your Angular 6 application
Docker as we know, is an open platform for developers and sysadmins to build, ship, and run distributed applications, whether on laptops, data center VMs, or the cloud. In this post, we are going to take look at how to build a Docker image for Angular application (typically the steps are the same for any type of application).
Quick Snapshot
Step #1. Setup Docker
- From the docker site, install the latest version of the docker for your platform. Docker is available in two editions: Community Edition (CE) and Enterprise Edition (EE). Docker Community Edition (CE) is ideal for developers and small teams looking to get started with Docker and experimenting with container-based apps. Docker Enterprise Edition (EE) is designed for enterprise development and IT teams who build, ship, and run business critical applications in production at scale.
- Once the installation of docker is over, check the installation by running following command
docker run hello-world
:Image – Validate Docker Installation - Run
docker --version
to check the version of the docker you’re running.Image – Check Docker version
OK, now we have got the docker setup, the next step is to define the docker container.
Step #2. Create Dockerfile for our container
-
- Dockerfile will define what goes on in the environment inside the container. Access to resources like networking interfaces and disk drives is virtualized inside this environment, which is isolated from the rest of the system, so you have to map ports to the outside world, and be specific about what files you want to “copy in” to that environment. So that you can expect that the build of your app defined in this Dockerfile will behave exactly the same wherever it runs.
- Common Dockerfile instructions start with RUN, ENV, FROM, MAINTAINER, ADD, and CMD, among others.
- FROM – Specifies the base image that the Dockerfile will use to build a new image. As an example, we are going to use phusion/baseimage as our base image (this is minimal Ubuntu-based image)
- MAINTAINER – Specifies the Dockerfile Author Name and his/her email.
- RUN – Runs any UNIX command to build the image.
- ENV – Sets the environment variables. For this post, JAVA_HOME is the variable that is set.
- CMD – provides the facility to run commands at the start of container. This can be overridden upon executing the docker run command.
- ADD – This instruction copies the new files, directories into the Docker container file system at a specified destination.
- EXPOSE – This instruction exposes a specified port to the host machine.
- To begin with, create a new folder and then create a file in it named “Dockerfile” with the following content.
# Dockerfile FROM httpd:2.4 MAINTAINER Author Name [email protected]
- Once we have the baseimage set,next step is to copy the Angular dist folder contents (assumption here is to already you have run the
ng build --prod
)#copy angular dist folder to container COPY dist/ /usr/local/apache2/htdocs/
- Next is to copy custom .htaccess and httpd.conf files to the container (as below)
#copy htaccess and httpd.conf to container COPY .htaccess /usr/local/apache2/htdocs/ COPY httpd.conf /usr/local/apache2/conf/httpd.conf
- Add below lines to .htaccess to add rewrite rules
RewriteEngine On # If an existing asset or directory is requested go to it as it is RewriteCond %{DOCUMENT_ROOT}%{REQUEST_URI} -f [OR] RewriteCond %{DOCUMENT_ROOT}%{REQUEST_URI} -d RewriteRule ^ - [L] # If the requested resource doesn't exist, use index.html RewriteRule ^ /index.html
- Add below lines to relevant sections of httpd.conf
LoadModule rewrite_module /usr/lib/apache2/modules/mod_rewrite.so LoadModule mpm_event_module modules/mod_mpm_event.so DocumentRoot "/usr/local/apache2/htdocs" <Directory "/usr/local/apache2/htdocs"> Options Indexes FollowSymLinks AllowOverride All Require all granted </Directory>
- Then coming back to Dockerfile, change the permissions
#change permissions RUN chmod -R 755 /usr/local/apache2/htdocs/
- Finally, add expose port command
#expose port EXPOSE 4200
- Docker file would now be looking like the one below
FROM httpd:2.4 #copy angular dist folder to container COPY dist/ /usr/local/apache2/htdocs/ #copy htaccess and httpd.conf to container COPY .htaccess /usr/local/apache2/htdocs/ COPY httpd.conf /usr/local/apache2/conf/httpd.conf #change permissions RUN chmod -R 755 /usr/local/apache2/htdocs/ #expose port EXPOSE 4200
Step #3. Build Docker Image
Now that we have completed Dockerfile, next step is to build Docker image by docker build command
docker build -f UIDockerfile -t angularapp .
Here -t
specifies the name of the image and -f
specifies the name of the Dockerfile. Image tag I have left it empty.

Congrats! You’ve successfully built a container for your Angular application.
Step #4. Test Docker Image
To run the Docker image, execute the following commands
docker run -p 4200:4200 angularapp
Here -p
specifies the port container:host mapping.
Launch your browser and hit http://localhost:4200
to access the application running on your container.

Step #5. Ngnix Dockerfile
If you want to use Ngnix instead of Apache then here is sample Dockerfile
FROM nginx COPY nginx.conf /etc/nginx/nginx.conf COPY dist/prod /usr/share/nginx/html
As you can see we are copying all the files from our dist
folder and we are also replacing the Nginx configuration with our own custom configuration (like the one below)
Ngnix sample configuration
server { listen 80; location / { root /usr/share/nginx/html; index index.html index.htm; try_files $uri $uri/ /index.html =404; } }
Congrats! You’ve successfully built and run container for your Angular application.
Step #6. Docker Compose Configuration
If you’re looking for running multi-container applications using Docker Compose tool then the configuration is as easy, there would be YAML file to configure your application’s services/networks/volumes, etc., Then, with a single command, you can create and start all the services from the compose configuration.
Here are the key steps :
- Define
Dockerfile
for your container(s). - Define
docker-compose.yml
for the services that make up your application services. - Run
docker-compose up
and Compose starts and runs your entire app.
Sample docker-compose.yml
for Angular UI application would look like this:
version: '3' services: angularui: build: context: . dockerfile: UIDockerfile ports: - '4200:4200' networks: - samplenet networks: samplenet: null
To scale services using Docker compose refer here. There is much more to the Docker platform than what was covered here, but now you would have got a good idea of the basics of building containers for an application.
Like this post? Don’t forget to share it!
Additional Resources:
- Official documentation as a reference to understand any command.
- Docker build reference
- Docker run reference
- Best Practices article on writing Docker files.
- Test your knowledge on Dockerfile.
- TOP 6 GUI tools for managing Docker environments
- Docker tutorial – Build Docker image for your Java application
- How to aggregate Docker Container logs and analyse with ELK stack ?
- Implementing secure containers using gVisor+Docker tutorial


Average Rating