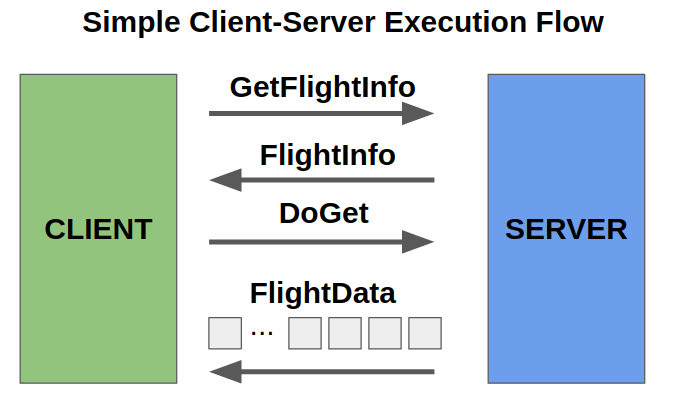
Unlock High-Performance Data Transfers with Apache Arrow Flight
In today’s data-driven world, fast, efficient data transfer is crucial for high-performance applications. Traditional methods, such as REST APIs or JDBC, often struggle with large datasets, leading to bottlenecks and high latency. That’s where Apache Arrow Flight comes in a high-performance, zero-copy data transfer framework built for speed and scalability.
In this article, we’ll explore how Apache Arrow Flight can revolutionize client-server data transfers in Java applications. We’ll guide you through setting up a basic client-server model using Apache Arrow Flight and highlight its performance benefits.
Quick Snapshot
What is Apache Arrow Flight?
Apache Arrow Flight is a cutting-edge framework that leverages the Apache Arrow columnar memory format and gRPC for high-speed, low-latency data transfers. It’s designed to address the limitations of traditional data transfer methods and is ideal for scenarios requiring the movement of large datasets, such as real-time analytics, machine learning, and big data processing.

Key Features of Apache Arrow Flight:
- Zero-Copy Data Transfer: Avoids serialization overhead by allowing direct memory access, leading to faster data transfer.
- High Throughput: Achieves impressive transfer rates, with benchmarks showing up to 6,000 MB/s for
DoGet()
operations and 4,800 MB/s forDoPut()
operations. - Cross-Language Compatibility: Supports multiple programming languages, including Java, Python, and C++.
- Built on gRPC: Utilizes gRPC for reliable and scalable communication, ensuring robust performance in distributed environments.
Setting Up Apache Arrow Flight in Java
To integrate Apache Arrow Flight into your Java applications, follow these easy steps to set up a client-server architecture. This will demonstrate how Arrow Flight can help you transfer large amounts of data quickly and efficiently.
1. Add Maven Dependencies
First, include the necessary dependencies in your pom.xml
to enable Arrow Flight in your Java project:
<dependencies>
<dependency>
<groupId>org.apache.arrow</groupId>
<artifactId>arrow-flight</artifactId>
<version>12.0.0</version>
</dependency>
<dependency>
<groupId>org.apache.arrow</groupId>
<artifactId>arrow-vector</artifactId>
<version>12.0.0</version>
</dependency>
</dependencies>
2. Set Up the Flight Server
Next, create a basic Flight server. This server will handle client requests and send data back using Arrow Flight. Implement the FlightProducer
interface to define how the data is transferred.
import org.apache.arrow.flight.*;
import org.apache.arrow.memory.RootAllocator;
public class SimpleFlightServer {
public static void main(String[] args) throws Exception {
BufferAllocator allocator = new RootAllocator(Long.MAX_VALUE);
Location location = Location.forGrpcInsecure("localhost", 12233);
FlightProducer producer = new NoOpFlightProducer(); // Replace with custom implementation
FlightServer server = FlightServer.builder(allocator, location, producer).build();
server.start();
System.out.println("Server started at " + location.getUri());
server.awaitTermination();
}
}
3. Create the Flight Client
Now, set up a simple client to interact with the server. The client will connect to the server and fetch data using Arrow Flight.
import org.apache.arrow.flight.*;
import org.apache.arrow.memory.RootAllocator;
public class SimpleFlightClient {
public static void main(String[] args) throws Exception {
BufferAllocator allocator = new RootAllocator(Long.MAX_VALUE);
Location location = Location.forGrpcInsecure("localhost", 12233);
FlightClient client = FlightClient.builder(allocator, location).build();
FlightInfo info = client.getInfo(FlightDescriptor.command("example"));
for (FlightEndpoint endpoint : info.getEndpoints()) {
try (FlightStream stream = client.getStream(endpoint.getTicket())) {
while (stream.next()) {
VectorSchemaRoot root = stream.getRoot();
// Process data
}
}
}
}
}
Performance Benchmarks: Why Apache Arrow Flight Outperforms Traditional Methods
Apache Arrow Flight leverage gRPC’s sophisticated “bidirectional” streaming feature, built on HTTP/2 streaming, enabling clients and servers to exchange data and metadata concurrently while processing requests.
Apache Arrow Flight isn’t just fast it’s significantly faster than traditional data transfer methods. Benchmark studies show:
- DoGet() Operations: Achieves up to 6,000 MB/s throughput.
- DoPut() Operations: Reaches 4,800 MB/s throughput.
- ODBC vs. Arrow Flight: Benchmarks demonstrate 20x to 30x faster performance with Arrow Flight compared to ODBC connections.
These impressive results make Apache Arrow Flight the ideal choice for applications that require high-speed data transfer, such as machine learning or big data processing.
Use Cases for Apache Arrow Flight
Apache Arrow Flight is perfect for scenarios that demand fast and reliable data exchange. Here are some key use cases:
- Real-Time Analytics: Ideal for applications that need to process large datasets on the fly and display results in real-time.
- Machine Learning Pipelines: Arrow Flight enables fast data ingestion into machine learning models, reducing time spent on data preprocessing.
- Big Data Processing: Whether in distributed systems or across data lakes, Arrow Flight simplifies the movement of large volumes of data between systems.
- ETL Workflows: With Arrow Flight, data transfer is faster, reducing bottlenecks in your extract, transform, and load processes.
Best Practices for Using Apache Arrow Flight
To make the most of Apache Arrow Flight, consider the following best practices:
- Efficient Memory Management: Be mindful of memory usage by managing the
RootAllocator
carefully to prevent leaks. - Close Resources: Always ensure that Flight streams and clients are properly closed to avoid resource wastage.
- Schema Consistency: Keep schemas consistent across client and server to avoid data mismatches.
- Security: Implement TLS and proper authentication mechanisms to secure your data transfers.
For more detailed information on using Arrow Flight, you can refer to the official Apache Arrow Flight documentation.
Conclusion: Maximize Data Transfer Efficiency with Apache Arrow Flight
Apache Arrow Flight provides an exceptional solution for high-performance data transfers, especially in Java-based applications. By leveraging the Arrow columnar format and gRPC, Arrow Flight minimizes latency and maximizes throughput, enabling real-time analytics, machine learning, and big data workflows.
If you’re ready to upgrade your data transfer capabilities and overcome the limitations of traditional methods, Apache Arrow Flight is the perfect tool to enhance your application’s performance.
Get started today and experience the speed and efficiency of Arrow Flight for yourself!
Resources:


Average Rating